After getting some general knowledge in the first tutorial, this second tutorial will be talking about variables, assignments and initializations.
A variable in Java has a type, name and a value. In the first tutorial we mostly talked about primitive data types. The non-primitive data types or also called reference data types are objects and arrays, which will be discussed in next tutorials.
We call variable a place in the computer’s memory where we can store data. Be careful; don’t name two variables with the same name! Each variable has a unique name.
Now let’s see how you can declare a variable.
ü A variable must be declared with a data type or an error will be generated!
ü You can declare a variable either inside the class curly brackets or inside the main method’s curly brackets.
ü Variable names can only contain letters, numbers, and the underscore ( _ ) symbol
ü Variable names can not start with numbers, only letters or the underscore ( _ ) symbol (but variable names can contain numbers)
Here is an example of how you declare an integer:
public class Var { public static void main(String[] args) { int var; } } |
When you want to declare more than 1 variable of the same type you can separate the names with a comma. For example:
public class Var { public static void main(String[] args) { int var, var2; } } |
Giving a variable a value when declared is called initialization. If you want to print variables on the screen, as mentioned in the previous tutorial you must use the println() method.
Here’s an example of an executable code:
import java.awt.*; public class Var { public static void main(String[] args) { int var = 10, var2=20;// initialization System.out.println("The value of the first number is: " + var + " and the value of the second number is: " + var2); } } |
In the output you will get:
The value of the first number is: 10 and the value of the second number is: 20
In Java, you use the keyword final to denote a constant. The keyword final indicates that you can assign to the variable once, then its value is set once and for all. Suppose you do this:
final int num = 14;
After this, nowhere in the code you can change the value of num.
Now, suppose you did not initialize the final integer ‘num’ while declaring it.
final int num;
num = 14; //This is fine
num= 11; //Compiler error
The ‘final’ keyword can be used for a class declaration or method declaration. We will explain this part in another tutorial.
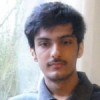
About Ali Gajani
Hi. I am Ali Gajani. I started Mr. Geek in early 2012 as a result of my growing enthusiasm and passion for technology. I love sharing my knowledge and helping out the community by creating useful, engaging and compelling content. If you want to write for Mr. Geek, just PM me on my Facebook profile.